Top 7 TypeScript Features Every Developer Should Know
Prashant Rayamajhi
@prashantrayamajhi
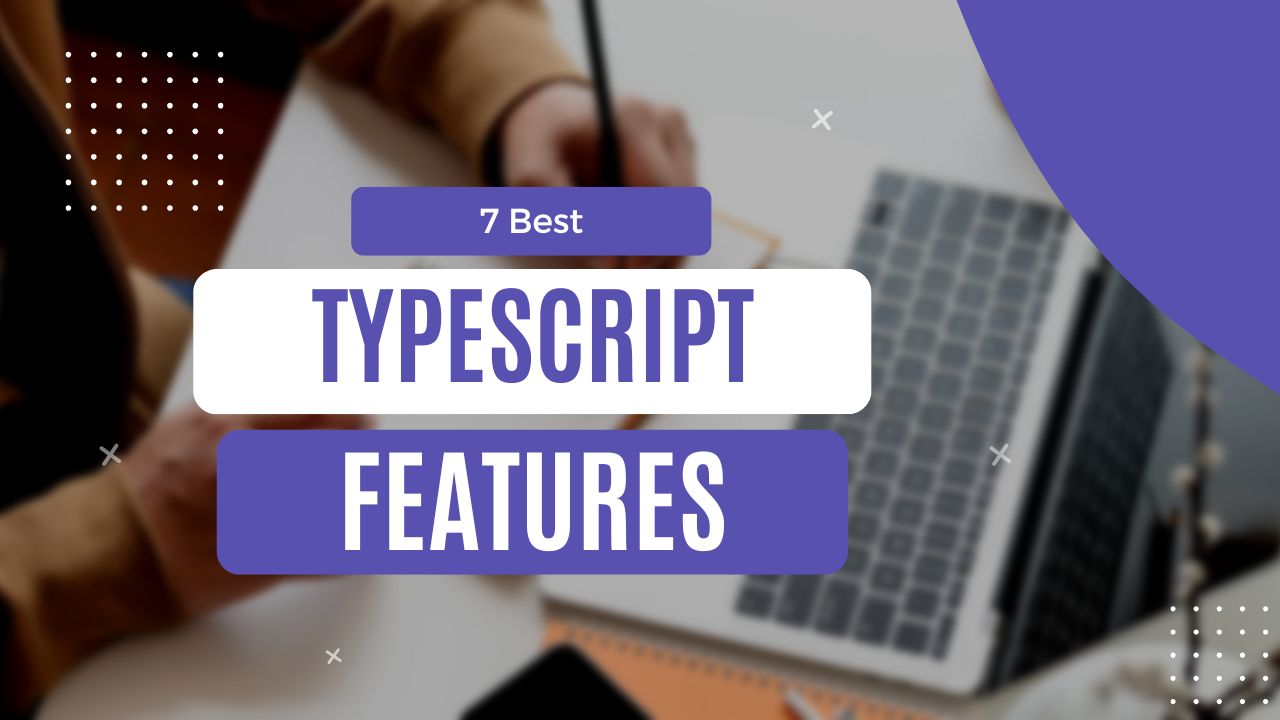
TypeScript has become a go-to choice for JavaScript developers, providing type safety and advanced tooling to enhance productivity. Here’s a breakdown of seven powerful TypeScript features that make it a favorite among developers.
1. Static Typing
TypeScript's static typing is one of its standout features. By defining types for variables, parameters, and return values, you get instant feedback on potential errors as you write code. For example:
let username: string = "JohnDoe";
username = 42; // Error: Type 'number' is not assignable to type 'string'.
Static typing not only catches mistakes early but also helps make the codebase more readable and maintainable.
2. Type Inference
TypeScript is smart enough to infer types without explicit annotations, making the code cleaner while still providing type safety.
let age = 30; // TypeScript infers 'age' as a number
Type inference allows you to write less code without compromising the safety and readability of your codebase.
3. Interfaces
Interfaces define the structure of an object, allowing you to define the shape and ensure objects adhere to a certain structure.
interface User {
name: string;
age: number;
isAdmin: boolean;
}
const newUser: User = {
name: "Alice",
age: 25,
isAdmin: true
};
Interfaces are invaluable for working with structured data and building scalable applications.
4. Union and Intersection Types
Union types allow variables to hold more than one type, while intersection types combine multiple types. These features are handy when working with multiple potential data shapes.
function printId(id: number | string) {
console.log("ID:", id);
}
type AdminUser = User & { isAdmin: boolean };
Unions and intersections make TypeScript more versatile, allowing you to handle complex data scenarios.
5. Enums
Enums let you define a set of named constants, making your code more readable and reducing the chances of invalid values.
enum Direction {
Up = "UP",
Down = "DOWN",
Left = "LEFT",
Right = "RIGHT"
}
let move: Direction = Direction.Up;
Enums are especially useful for managing state and providing meaningful values in your code.
6. Generics
Generics allow you to create reusable components and functions by accepting variable types, enhancing flexibility without losing type safety.
function identity<T>(value: T): T {
return value;
}
identity<number>(42); // 42
identity<string>("Hello"); // "Hello"
Generics bring the power of type-safe, reusable components, making your code more modular and maintainable.
7. Decorators
Decorators are a powerful feature often used in frameworks like Angular. They allow you to add metadata to classes and methods, making it easier to implement features like dependency injection.
function Log(target: any, propertyKey: string, descriptor: PropertyDescriptor) {
console.log(`${propertyKey} was called`);
}
class Person {
@Log
sayHello() {
console.log("Hello!");
}
}
Decorators make it easier to add reusable functionality to your code in a concise, declarative way.